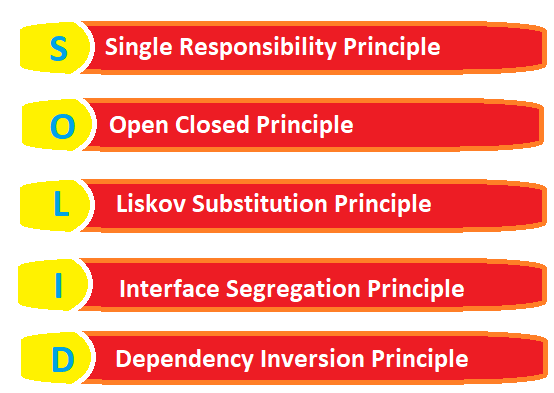
Learning SOLID Principles C# with Examples : Best Practices, Applying SOLID principles in c# to write better C# code
Introduction
solid principles in c# : As a software developer, writing code that is not only functional but also maintainable and scalable is crucial. This is where SOLID principles c# with example come into play. SOLID is an acronym for five essential design principles that help developers create robust, flexible, and maintainable code.In the upcoming blog post, we will delve into practical code examples in C# to comprehensively understand each of these principles. By the conclusion of this exploration, you’ll possess a profound grasp of effectively implementing these essential guidelines within your own projects.
Unlock the potential of software design with the Factory Design Pattern in C#, revolutionizing efficient object creation and code flexibility.
Table of Contents
What are SOLID Principles? (Detail understanding of Solid Principles c# with Example)
SOLID principles were introduced by Robert C. Martin, also known as Uncle Bob, and have become a cornerstone of object-oriented design. Let’s briefly go through each principle:
Explore the top 5 GPS tracker devices for efficient tracking and location services.
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) is one of the fundamental principles in object-oriented programming and software design. It is one of the five SOLID principles introduced by Robert C. Martin, aimed at improving the design and maintainability of software systems. The SRP is often summarized as follows:
“A class should have only one reason to change.”
In essence, the SRP advocates that a software module, typically represented by a class in object-oriented programming, should have a single and well-defined responsibility or job. This means that the module should encapsulate and be responsible for one specific aspect of the overall functionality of the system. When a class adheres to the SRP, it becomes focused, easier to understand, and simpler to maintain.
Key aspects and implications of the Single Responsibility Principle:
- Clear Responsibility: Each class or module should have a clear, concise, and singular purpose or responsibility. This makes it easier to understand the code’s intent.
- Encapsulation: SRP promotes encapsulation by ensuring that each class has a well-defined area of concern. This helps in isolating changes within that class, minimizing the risk of unexpected side effects.
- High Cohesion: A class adhering to SRP tends to have high cohesion, meaning that its methods and attributes are closely related and work together to accomplish its singular task.
- Low Coupling: By isolating responsibilities, SRP reduces the dependencies between classes. Low coupling makes the codebase more flexible and maintainable, as changes to one class are less likely to impact other parts of the system.
- Easier Testing: Classes following SRP are often easier to test because their responsibilities are well-defined. This allows for more targeted and thorough unit testing.
- Scalability: SRP contributes to the scalability of the codebase. As new requirements emerge, it’s often easier to extend or modify a class with a single responsibility rather than trying to retrofit an existing, multifaceted class.
Example:
Let’s consider a scenario where we have a Customer
class that handles both customer information and sending emails. This violates the SRP, as it has two distinct responsibilities.
Before SRP:
class Customer
{
public string Name { get; set; }
public string Email { get; set; }
public void SaveCustomerInfo()
{
// Save customer information to the database
}
public void SendEmail()
{
// Send a welcome email to the customer
}
}
After applying SRP:
class Customer
{
public string Name { get; set; }
public string Email { get; set; }
}
class CustomerRepository
{
public void SaveCustomerInfo(Customer customer)
{
// Save customer information to the database
}
}
class EmailService
{
public void SendEmail(Customer customer)
{
// Send a welcome email to the customer
}
}
By separating the responsibilities into distinct classes (CustomerRepository
for data storage and EmailService
for sending emails), we adhere to the SRP. Now, changes related to data storage won’t impact email sending, and vice versa, leading to a more maintainable and flexible codebase.
In summary, the Single Responsibility Principle is a guiding principle in software design that encourages the creation of small, focused, and modular classes, each with a clearly defined responsibility. This leads to more maintainable, flexible, and understandable software systems, which are easier to develop, test, and extend as the project evolves.
2. Open/Closed Principle (OCP)
The Open/Closed Principle (OCP) is one of the five SOLID principles of object-oriented software design, first introduced by Bertrand Meyer. The OCP is a fundamental concept aimed at promoting software systems that are both adaptable and maintainable. It can be stated as follows:
“Software entities (e.g., classes, modules, functions) should be open for extension but closed for modification.”
In essence, the OCP suggests that once a software component (such as a class) is written and functioning correctly, it should not be altered to accommodate new features or changes in behavior. Instead, the system should allow for the extension of existing components or the creation of new ones to add new functionality.
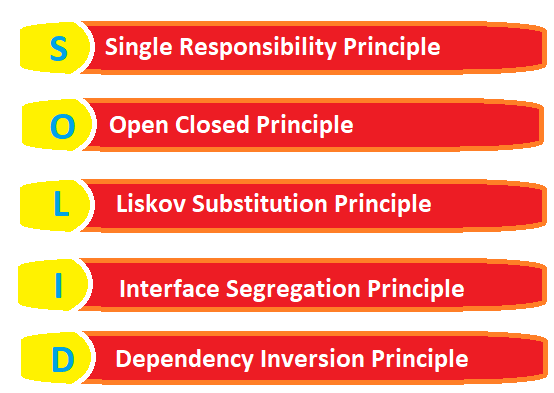
Key aspects and implications of the Open/Closed Principle:
- Open for Extension: This part of the principle implies that you should be able to add new features or behaviors to a system without having to modify the existing code. This is typically achieved through techniques like inheritance, interfaces, or abstract classes.
- Closed for Modification: Once a class is designed and implemented to fulfill its specific responsibility, it should remain unchanged. This helps in preserving the stability of existing code and minimizes the risk of introducing new bugs when making changes.
- Abstraction: To follow OCP, you often use abstraction mechanisms like interfaces or abstract classes to define contracts or APIs that can be extended by implementing new classes. This allows you to add new behavior by creating new classes that adhere to the same interface.
- Reduced Risk: By adhering to OCP, you reduce the risk of introducing defects into existing code because you’re not modifying it. New features are added through new code, which can be developed and tested independently.
- Ease of Maintenance: Code that adheres to OCP is typically easier to maintain because changes are isolated to specific extensions rather than being scattered throughout the existing codebase.
- Flexibility: OCP makes your software more flexible and adaptable to changing requirements. New functionality can be added without affecting the existing code, making it easier to respond to evolving user needs.
Example:
Let’s say we have a simple Shape
class hierarchy representing various shapes like circles and squares. We want to calculate the area of these shapes while adhering to the OCP.
Before OCP:
class Shape
{
public virtual double CalculateArea()
{
return 0;
}
}
class Circle : Shape
{
public double Radius { get; set; }
public override double CalculateArea()
{
return Math.PI * Radius * Radius;
}
}
class Square : Shape
{
public double SideLength { get; set; }
public override double CalculateArea()
{
return SideLength * SideLength;
}
}
In the above code, adding a new shape (e.g., Triangle
) would require modifying the Shape
class, violating the OCP.
After applying OCP:
interface IShape
{
double CalculateArea();
}
class Circle : IShape
{
public double Radius { get; set; }
public double CalculateArea()
{
return Math.PI * Radius * Radius;
}
}
class Square : IShape
{
public double SideLength { get; set; }
public double CalculateArea()
{
return SideLength * SideLength;
}
}
class Triangle : IShape
{
public double Base { get; set; }
public double Height { get; set; }
public double CalculateArea()
{
return 0.5 * Base * Height;
}
}
By introducing the IShape
interface and implementing it in each shape class, we’ve adhered to the OCP. Now, we can add new shapes by creating new classes that implement IShape
, without modifying the existing code. This promotes code reusability and maintains the stability of the existing codebase while allowing for seamless extension.
In summary, the Open/Closed Principle advocates designing software components to be open for extension, allowing for the addition of new features or behaviors without modifying existing code, while simultaneously being closed for modification to maintain the stability of the existing system. This principle encourages the use of abstraction and object-oriented techniques to achieve a more flexible and maintainable codebase.
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) is one of the SOLID principles of object-oriented programming and software design, introduced by Barbara Liskov in 1987. The LSP is a guideline that helps ensure that derived or subclassed classes can be used interchangeably with their base or parent classes without affecting the correctness of the program. It can be succinctly stated as follows:
“Objects of a subclass should be able to replace objects of the base class without affecting the correctness of the program.”
In essence, the LSP emphasizes that a derived class should adhere to the contract established by its base class. This means that a subclass should not introduce new behaviors that are not compatible with the behavior of the base class, and it should not override or alter the functionality of the base class in a way that breaks the expected behavior of the program.
Key aspects and implications of the Liskov Substitution Principle:
- Behavioral Compatibility: Subclasses should extend the behavior of their base classes in a way that is consistent and compatible with the base class. This ensures that clients using the base class can rely on the same behavior when working with subclasses.
- Method Signatures: Subclasses should maintain the same method signatures as their base classes, including method names, parameters, and return types. This ensures that client code can call methods on subclasses in the same way as on the base class.
- Inheritance vs. Interface: LSP is applicable to both class inheritance and interface implementation. Subclasses should conform to the interface or abstract class they inherit from and should not violate the expected behavior of the base class.
- Preconditions and Postconditions: Subclasses should meet the preconditions (requirements) and postconditions (guarantees) of their base class’s methods. This ensures that using a subclass in place of the base class does not lead to unexpected failures or violations of program logic.
- Exception Handling: Subclasses should not throw exceptions that are not part of the base class’s contract. If the base class does not specify exceptions, subclasses should avoid throwing new exceptions that clients are not prepared to handle.
- Design by Contract: LSP aligns with the broader concept of “Design by Contract,” where classes define clear expectations (contracts) for their behavior, and subclasses must honor these contracts.
Discover a wealth of C# interview questions by visiting our page on C# interview questions: C# Interview Questions.
Example:
Consider a scenario where we have a Bird
base class and two derived classes, Sparrow
and Ostrich
. We want to ensure that the LSP is followed, meaning that instances of derived classes can be used interchangeably with instances of the base class.
Before LSP:
class Bird
{
public virtual void Fly()
{
Console.WriteLine("Flying...");
}
}
class Sparrow : Bird
{
public override void Fly()
{
Console.WriteLine("Sparrow flying...");
}
}
class Ostrich : Bird
{
public override void Fly()
{
throw new NotSupportedException("Ostriches cannot fly.");
}
}
In the above code, the Ostrich
class violates the LSP because it throws an exception when trying to perform a fundamental behavior of the base class.
After applying LSP:
interface IBird
{
void Fly();
}
class Bird : IBird
{
public virtual void Fly()
{
Console.WriteLine("Flying...");
}
}
class Sparrow : IBird
{
public void Fly()
{
Console.WriteLine("Sparrow flying...");
}
}
class Ostrich : IBird
{
public void Fly()
{
Console.WriteLine("Ostrich cannot fly.");
}
}
By introducing the IBird
interface and ensuring that all derived classes implement the Fly
method without violating their inherent characteristics, we’ve followed the LSP. Now, instances of Sparrow
and Ostrich
can be used interchangeably with instances of the Bird
base class, without causing unexpected behavior or exceptions.
The Liskov Substitution Principle promotes a strong and consistent behavior across inheritance hierarchies, enhancing the reliability and maintainability of your codebase.
In summary, the Liskov Substitution Principle emphasizes the importance of maintaining behavioral compatibility between base and derived classes to ensure that objects of derived classes can seamlessly replace objects of the base class without causing errors or unexpected behavior. Adhering to this principle promotes robust, maintainable, and extensible object-oriented software design.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP) is one of the SOLID principles of object-oriented programming and software design, introduced as part of Robert C. Martin’s principles for writing maintainable and scalable code. The ISP focuses on the design of interfaces and aims to ensure that interfaces are small, focused, and cater to the specific needs of clients or classes that implement them. It can be stated as follows:
“Clients should not be forced to depend on interfaces they do not use.”
In essence, the ISP suggests that when defining interfaces, they should contain only the methods that are relevant to the implementing classes. Classes that implement these interfaces should not be burdened with having to provide implementations for methods they don’t need. This promotes a more modular and flexible design, making it easier to develop and maintain software systems.
Key aspects and implications of the Interface Segregation Principle:
- Client-Specific Interfaces: Interfaces should be tailored to the specific requirements of the client classes that implement them. This means that each client should have its own interface with methods that are relevant to its needs.
- Avoid Fat Interfaces: Avoid creating “fat” interfaces that contain a large number of methods. Fat interfaces force implementing classes to provide implementations for all methods, even if they don’t need them, which can lead to unnecessary code complexity.
- Granular Interfaces: Break down interfaces into smaller, more granular pieces. This allows clients to implement only the interfaces or methods that are directly related to their functionality.
- Default Implementations: In languages that support it (e.g., Java 8 and later), you can provide default implementations for interface methods. This can help minimize the burden on implementing classes while allowing them to override methods as needed.
- Dependency Management: By adhering to ISP, you can reduce unnecessary dependencies between classes and modules. Clients only depend on the interfaces they use, which leads to a more modular and maintainable codebase.
- Ease of Testing: Smaller, focused interfaces often result in classes that are easier to test in isolation because you can provide mock implementations of the required interfaces without having to implement irrelevant methods.
Example:
Imagine a scenario where we’re designing a printer system with different types of printers and their functionalities. Let’s explore how to apply ISP to avoid fat interfaces.
Before ISP:
interface IPrinter
{
void Print();
void Scan();
void Fax();
}
class LaserPrinter : IPrinter
{
public void Print()
{
// Implement print for laser printer
}
public void Scan()
{
// Implement scan for laser printer
}
public void Fax()
{
// Implement fax for laser printer
}
}
class InkjetPrinter : IPrinter
{
public void Print()
{
// Implement print for inkjet printer
}
public void Scan()
{
// Implement scan for inkjet printer
}
public void Fax()
{
// Implement fax for inkjet printer
}
}
In the above code, both LaserPrinter
and InkjetPrinter
are forced to implement methods they don’t need (Scan
and Fax
) due to the fat IPrinter
interface, which violates ISP.
After applying ISP:
interface IPrinter
{
void Print();
}
interface IScanner
{
void Scan();
}
interface IFaxMachine
{
void Fax();
}
class LaserPrinter : IPrinter, IScanner
{
public void Print()
{
// Implement print for laser printer
}
public void Scan()
{
// Implement scan for laser printer
}
}
class InkjetPrinter : IPrinter, IFaxMachine
{
public void Print()
{
// Implement print for inkjet printer
}
public void Fax()
{
// Implement fax for inkjet printer
}
}
By segregating the interfaces into IPrinter
, IScanner
, and IFaxMachine
, we adhere to ISP. Now, each class only implements the methods that are relevant to its functionality, resulting in cleaner and more maintainable code. Clients can use the specific interfaces they need, promoting a more flexible and focused design.
In summary, the Interface Segregation Principle advocates the creation of small, client-specific interfaces that contain only the methods relevant to the implementing classes. This approach promotes modularity, flexibility, and maintainability in software design by reducing unnecessary dependencies and ensuring that clients are not forced to implement methods they don’t need.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) is one of the SOLID principles of object-oriented programming and software design, introduced by Robert C. Martin. The DIP is a guideline that emphasizes decoupling between high-level modules and low-level modules, promoting a more flexible and maintainable design. It can be summarized as follows:
“High-level modules should not depend on low-level modules. Both should depend on abstractions (interfaces or abstract classes).”
In essence, the DIP encourages designing software systems in a way that higher-level components (e.g., classes or modules representing business logic) are not directly dependent on lower-level components (e.g., classes responsible for specific implementations or details). Instead, both high-level and low-level components should depend on abstractions or interfaces, allowing for interchangeable implementations and reducing the risk of tight coupling.
Key aspects and implications of the Dependency Inversion Principle:
- Abstraction Layer: Introduce an abstraction layer, typically in the form of interfaces or abstract classes, that defines the contract or API that high-level modules expect from low-level modules. This abstraction acts as a mediator between the two.
- Inversion of Control (IoC): The DIP often goes hand-in-hand with the IoC principle. In IoC, the control of object creation and the flow of dependencies is shifted from the high-level modules to a container or framework. This allows for dynamic wiring of dependencies and makes it easier to swap implementations.
- Flexibility: By depending on abstractions rather than concrete implementations, high-level modules become more flexible and can work with different low-level modules that adhere to the same abstraction. This makes it easier to extend and modify the system.
- Testing: The use of abstractions makes it simpler to create mock or stub implementations for testing purposes. High-level modules can be tested in isolation from their concrete dependencies.
- Reduced Coupling: DIP helps reduce tight coupling between components, making the codebase more maintainable. Changes to low-level implementations have minimal impact on high-level modules.
- Plug-and-Play: DIP facilitates a “plug-and-play” approach, where new implementations can be added or existing ones replaced without requiring significant modifications to the high-level modules.
Example:
Let’s consider a simple logging system where we want to log messages to different destinations (e.g., file, database) while adhering to the DIP.
Before DIP:
enum LogDestination
{
File,
Database
}
class Logger
{
private LogDestination _destination;
public Logger(LogDestination destination)
{
_destination = destination;
}
public void Log(string message)
{
switch (_destination)
{
case LogDestination.File:
// Log to file
break;
case LogDestination.Database:
// Log to database
break;
}
}
}
In the above code, the Logger
class directly depends on the specific implementations of logging to a file or a database, violating the DIP.
After applying DIP:
interface ILogWriter
{
void WriteLog(string message);
}
class FileLogWriter : ILogWriter
{
public void WriteLog(string message)
{
// Implement writing log to a file
}
}
class DatabaseLogWriter : ILogWriter
{
public void WriteLog(string message)
{
// Implement writing log to a database
}
}
class Logger
{
private ILogWriter _logWriter;
public Logger(ILogWriter logWriter)
{
_logWriter = logWriter;
}
public void Log(string message)
{
_logWriter.WriteLog(message);
}
}
The motion sensor light automatically illuminates when it detects movement, providing convenience and enhanced security.
By introducing the ILogWriter
interface and implementing it in FileLogWriter
and DatabaseLogWriter
, we adhere to the DIP. The Logger
class now depends on the abstraction ILogWriter
rather than specific implementations. This allows for easy extension with new logging destinations without modifying the Logger
class, promoting a more flexible and maintainable design.
The Dependency Inversion Principle helps reduce tight coupling between components, making your codebase more modular and enhancing its testability and maintainability.
In summary, the Dependency Inversion Principle encourages the use of abstractions to decouple high-level modules from low-level modules, resulting in more flexible, maintainable, and extensible software systems. This principle promotes the idea that the relationships between components should be defined by abstract contracts rather than concrete implementations, allowing for greater adaptability and ease of development.
Conclusion
Understanding and applying the SOLID principles in your codebase can significantly improve the quality, maintainability, and scalability of your software projects. By following these best practices, you can write code that is easier to understand, extend, and modify, leading to a more efficient and productive development process. As you continue your journey as a software developer, keep these principles in mind and leverage them to build robust and reliable applications.
FAQs
What are the SOLID principles in c#?
The SOLID principles are a set of five design principles (SRP, OCP, LSP, ISP, and DIP) that guide developers in writing maintainable and flexible code.
How does the Single Responsibility Principle benefit software development?
The Single Responsibility Principle ensures that each class has a single responsibility, leading to cleaner and more maintainable code.
What is the Liskov Substitution Principle?
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the program’s correctness.
How can developers apply the Dependency Inversion Principle in C# projects?
Developers can apply the Dependency Inversion Principle by depending on abstractions (interfaces) rather than concrete implementations.
What are the advantages of adhering to SOLID principles?
Adhering to SOLID principles leads to code that is easier to understand, maintain, and extend, resulting in a more efficient and reliable software development process.
Related Post
- LINQ Join with Multiple Conditions: Mastering Advanced Queries
- Exploring C# Record vs Class: Making Informed Choices
- Mediator pattern c# example (Mediator design pattern c#)
- Understanding of What is Middleware in C# (.Net Core)
- Check out whats new features are coming in .Net 9
- Value Type and Reference Type in C# – A Guide to Value vs. Reference Types in C#
- Singleton Design Pattern in C# with Example A Step-by-Step Guide to Implementing the Singleton Design Pattern in C#
- Mastering the Factory Design Pattern in c# :Benefits of Using the Factory Design Pattern in C#
- Learning SOLID Principles C# with Examples : Best Practices, Applying SOLID principles in c# to write better C# code


2 Comments
replica hermes backpacks
Keep this going please, great job!
staten islander news org
We may pretty much all make use of learning even more about themselves and our health and wellness.
Certain activities and animation amounts can easily possess wonderful advantage to us, and all of us need to learn more information about them.
Your weblog seems to have provided helpful data that is useful to a number
of cultures and individuals, and I enjoy your writing your knowledge this way.